2022F_TT2
Question 1
Completion of this question demonstrates competency in user I/O and competency + mastery in variables
Your job is to write a simple booking app that asks the user to input a day of the week, a starting time, and duration. If the room is free during that time, it will be booked, if the room is not free for some or all of that time, the system will tell the user that the room could not be booked. You may assume that all times begin on a specific hour, and that all bookings are in hour-long increments.
In order to store the bookings, you should create a list of lists of booleans. That is to say, a list of seven elements where each element is a list of 24 boolean (True or False) values representing the booking status for a specific day/hour.
列表的整数倍增加;
lst = [1] print(lst * 20)
字符串内置 strip():去掉字符串的前后空格
s = " sunday " new_s = s.strip() print(s) print(new_s)
判断元素在不在序列当中
In [1]: days_of_week = { ...: "monday": 0, ...: "tuesday": 1, ...: "wednesday": 2, ...: "thursday": 3, ...: "friday": 4, ...: "saturday": 5, ...: "sunday": 6, ...: } In [2]: 1 in days_of_week Out[2]: False In [3]: "monday" in days_of_week Out[3]: True In [4]: lst = [1, 2, 3, "aiyc"] In [5]: 1 in lst Out[5]: True In [6]: 300 not in lst Out[6]: True
字符串转换问题
s = "aiyuechuang" print(s.isdigit()) s = "12112121212" print(s.isdigit()) s = "12112121212 " print(s.isdigit())
注意⚠️:包含开始时间,无需取到 duration!(开区间)
待一个小时的情况
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 1 start_time = 14 week = 1 print(bookings[week][14]) # 判断 14:00 本身是否空闲 # print(bookings[week][14 + 1])
待两个小时的情况
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 2 start_time = 14 week = 1 print(bookings[week][14]) # 判断 14:00 本身是否空闲 print(bookings[week][14 + 1]) # 判断第一个小时是否空闲 print(bookings[week][14 + 1 + 1]) # 判断第二个小时是否空闲
待三个小时的情况
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 3 # 待三个小时 start_time = 14 week = 1 print(bookings[week][14]) print(bookings[week][14 + 1]) # 判断第一个小时是否空闲 print(bookings[week][14 + 1 + 1]) # 判断第二个小时是否空闲 # print(bookings[week][14 + 1 + 1 + 1]) # 判断第三个小时是否空闲
Step 1:待三个小时以上的情况
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 3 # 待三个小时 start_time = 14 week = 1 # 判断三个小时 or 三个小时以上,我如下执行 print(bookings[week][14]) print(bookings[week][14 + 1]) # 判断第一个小时是否空闲 print(bookings[week][14 + 2]) # 判断第二个小时是否空闲 # print(bookings[week][14 + 3]) # 判断第三个小时是否空闲
Step 2:待三个小时以上的情况
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 3 # 待三个小时 start_time = 14 week = 1 # 判断三个小时 or 三个小时以上,我如下执行 print(bookings[week][14]) print(bookings[week][start_time + 1]) # 判断第一个小时是否空闲 print(bookings[week][start_time + 2]) # 判断第二个小时是否空闲 # print(bookings[week][start_time + 3]) # 判断第三个小时是否空闲
Step 3:待三个小时以上的情况
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 3 # 待三个小时 start_time = 14 week = 1 print(bookings[week][start_time + 0]) # 可以制作一个 +0 (类似数学当中构造一个:规律 or 公式)类似数学几何画辅助线 print(bookings[week][start_time + 1]) print(bookings[week][start_time + 2]) # print(bookings[week][start_time + 3])
Step 4:如何得到0~3?
for i in range(3): print(i) # ---output--- 0 1 2
Step 5:
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 3 # 待三个小时 start_time = 14 week = 1 # 如何得到0~3? for i in range(3): print(bookings[week][start_time + i])
Step 6:
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24] duration = 3 # 待三个小时 start_time = 14 week = 1 # 如何得到0~3? for i in range(duration): print(bookings[week][start_time + i])
lst = ["a", "b", "c"]
lst[0] = 100
print(lst) # 输出:[100, 'b', 'c']
lst = [['a', 'b'], ['c', 'd']]
# 把 'c' 改成 'Hello'
select1 = lst[1]
# select2 = select1[0]
select1[0] = 'Hello'
# print(select2)
print(lst)
lst = [['a', 'b'], ['c', 'd']]
lst[1][0] = 'Hello'
print(lst)
# -*- coding: utf-8 -*-
# @Time : 2024/11/16 09:03
# @Author : AI悦创
# @FileName: lo.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
# code is far away from bugs with the god animal protecting
# I love animals. They taste delicious.
# 初始化一周的预订状态,7天,每天24小时,默认False表示未预订
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24]
days_of_week = {
"monday": 0,
"tuesday": 1,
"wednesday": 2,
"thursday": 3,
"friday": 4,
"saturday": 5,
"sunday": 6,
}
# print(days_of_week['monday'])
# 输入星期几
day = input("请输入星期几(如:monday,tuesday等):").strip().lower()
if day not in days_of_week:
print("输入的星期不正确,程序结束。")
else:
day_index = days_of_week[day]
# 输入起始时间
start_time = input("请输入起始时间(24小时制,0-23之间的整数):").strip()
# if start_time.isdigit():
# pass
# else:
# print("时间格式不对")
if not start_time.isdigit() or int(start_time) < 0 or int(start_time) > 23:
print("输入的时间不在有效范围内,程序结束。")
else:
start_time = int(start_time)
# 输入持续时间
duration = input("请输入持续时间(小时,1-24之间的整数):").strip()
if not duration.isdigit() or int(duration) < 1 or int(duration) > 24 or start_time + int(duration) > 24:
print("持续时间不在有效范围内,程序结束。")
else:
duration = int(duration)
# 检查时间段是否可用
can_book = True # 先默认可以预定
for i in range(duration):
if bookings[day_index][start_time + i]:
can_book = False # 不能居住
break
if can_book == True:
# 更新预定状态
for i in range(duration):
bookings[day_index][start_time + i] = True # 列表修改值
print(f"预订成功!{day.capitalize()}从{start_time}:00到{start_time + duration}:00已预订。")
else:
print(f"预订失败!{day.capitalize()}从{start_time}:00到{start_time + duration}:00时间段已有预订。")
# -*- coding: utf-8 -*-
# @Time : 2024/11/16 09:03
# @Author : AI悦创
# @FileName: lo.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
# code is far away from bugs with the god animal protecting
# I love animals. They taste delicious.
# 初始化一周的预订状态,7天,每天24小时,默认False表示未预订
bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24]
days_of_week = {
"monday": 0,
"tuesday": 1,
"wednesday": 2,
"thursday": 3,
"friday": 4,
"saturday": 5,
"sunday": 6,
}
# print(days_of_week['monday'])
# 输入星期几
day = input("请输入星期几(如:monday,tuesday等):")
day_index = days_of_week[day]
# 输入起始时间
start_time = int(input("请输入起始时间(24小时制,0-23之间的整数):"))
duration = int(input("请输入持续时间(小时,1-24之间的整数):"))
# 检查时间段是否可用
can_book = True # 先默认可以预定
for i in range(duration):
if bookings[day_index][start_time + i]:
can_book = False # 不能居住
break
if can_book == True:
# 更新预定状态
for i in range(duration):
bookings[day_index][start_time + i] = True # 列表修改值
print(f"预订成功!{day.capitalize()}从{start_time}:00到{start_time + duration}:00已预订。")
else:
print(f"预订失败!{day.capitalize()}从{start_time}:00到{start_time + duration}:00时间段已有预订。")
# -*- coding: utf-8 -*-
# @Time : 2024/11/16 09:03
# @Author : AI悦创
# @FileName: lo.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
# code is far away from bugs with the god animal protecting
# I love animals. They taste delicious.
# 初始化一周的预订状态,7天,每天24小时,默认False表示未预订
# bookings = [[False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24, [False] * 24]
bookings = []
for i in range(7):
bookings.append([False] * 24)
days_of_week = {
"monday": 0,
"tuesday": 1,
"wednesday": 2,
"thursday": 3,
"friday": 4,
"saturday": 5,
"sunday": 6,
}
while True:
day = input("请输入星期几(如:monday,tuesday等,输入'exit'退出):")
if day == "exit":
break
day_index = days_of_week[day]
# 输入起始时间
start_time = int(input("请输入起始时间(24小时制,0-23之间的整数):"))
duration = int(input("请输入持续时间(小时,1-24之间的整数):"))
# 检查时间段是否可用
can_book = True # 先默认可以预定
for i in range(duration):
if bookings[day_index][start_time + i]:
can_book = False # 不能居住
break
if can_book == True:
# 更新预定状态
for i in range(duration):
bookings[day_index][start_time + i] = True # 列表修改值
print(f"预订成功!{day.capitalize()}从{start_time}:00到{start_time + duration}:00已预订。")
else:
print(f"预订失败!{day.capitalize()}从{start_time}:00到{start_time + duration}:00时间段已有预订。")
# -*- coding: utf-8 -*-
# @Time : 2024/11/16 09:03
# @Author : AI悦创
# @FileName: lo.py
# @Software: PyCharm
# @Blog :https://bornforthis.cn/
# code is far away from bugs with the god animal protecting
# I love animals. They taste delicious.
# 初始化一周的预订状态,7天,每天24小时,默认False表示未预订
bookings = []
for i in range(7):
bookings.append([False] * 24)
days_of_week = {
"monday": 0,
"tuesday": 1,
"wednesday": 2,
"thursday": 3,
"friday": 4,
"saturday": 5,
"sunday": 6,
}
# print(days_of_week['monday'])
# 输入星期几
while True:
day = input("请输入星期几(如:monday,tuesday等):").strip().lower()
if day == "exit":
break
if day not in days_of_week:
print("输入的星期不正确,程序结束。")
else:
day_index = days_of_week[day]
# 输入起始时间
start_time = input("请输入起始时间(24小时制,0-23之间的整数):").strip()
# if start_time.isdigit():
# pass
# else:
# print("时间格式不对")
if not start_time.isdigit() or int(start_time) < 0 or int(start_time) > 23:
print("输入的时间不在有效范围内,程序结束。")
else:
start_time = int(start_time)
# 输入持续时间
duration = input("请输入持续时间(小时,1-24之间的整数):").strip()
if not duration.isdigit() or int(duration) < 1 or int(duration) > 24 or start_time + int(duration) > 24:
print("持续时间不在有效范围内,程序结束。")
else:
duration = int(duration)
# 检查时间段是否可用
can_book = True # 先默认可以预定
for i in range(duration):
if bookings[day_index][start_time + i]:
can_book = False # 不能居住
break
if can_book == True:
# 更新预定状态
for i in range(duration):
bookings[day_index][start_time + i] = True # 列表修改值
print(f"预订成功!{day.capitalize()}从{start_time}:00到{start_time + duration}:00已预订。")
else:
print(f"预订失败!{day.capitalize()}从{start_time}:00到{start_time + duration}:00时间段已有预订。")
# 初始化一周的预订状态,7天,每天24小时,默认False表示未预订
bookings = [[False] * 24 for _ in range(7)]
# 定义星期和索引映射
days_of_week = {
"monday": 0,
"tuesday": 1,
"wednesday": 2,
"thursday": 3,
"friday": 4,
"saturday": 5,
"sunday": 6
}
# 输入星期几
day = input("请输入星期几(如:monday, tuesday等):").strip().lower()
if day not in days_of_week:
print("输入的星期不正确,程序结束。")
else:
day_index = days_of_week[day]
# 输入起始时间
start_time = input("请输入起始时间(24小时制,0-23之间的整数):").strip()
if not start_time.isdigit() or int(start_time) < 0 or int(start_time) > 23:
print("输入的时间不在有效范围内,程序结束。")
else:
start_time = int(start_time)
# 输入持续时间
duration = input("请输入持续时间(小时,1-24之间的整数):").strip()
if not duration.isdigit() or int(duration) < 1 or int(duration) > 24 or start_time + int(duration) > 24:
print("持续时间不在有效范围内,程序结束。")
else:
duration = int(duration)
# 检查时间段是否可用
can_book = True
for i in range(duration):
if bookings[day_index][start_time + i]:
can_book = False
break
if can_book:
# 更新预订状态
for i in range(duration):
bookings[day_index][start_time + i] = True
print(f"预订成功!{day.capitalize()}从{start_time}:00到{start_time + duration}:00已预订。")
else:
print(f"预订失败!{day.capitalize()}从{start_time}:00到{start_time + duration}:00时间段已有预订。")
# 初始化一周的预订状态,7天,每天24小时,默认False表示未预订
bookings = [[False] * 24 for _ in range(7)]
# 定义星期和索引映射
days_of_week = {
"monday": 0,
"tuesday": 1,
"wednesday": 2,
"thursday": 3,
"friday": 4,
"saturday": 5,
"sunday": 6
}
print("\n欢迎使用预订系统!")
while True:
print("\n请输入需要预订的信息:")
# 输入星期几
day = input("请输入星期几(如:monday, tuesday等,输入'exit'退出):").strip().lower()
if day == 'exit':
print("感谢使用预订系统!")
break
if day not in days_of_week:
print("输入的星期不正确,请重新输入!")
continue
day_index = days_of_week[day]
# 输入起始时间
start_time = input("请输入起始时间(24小时制,0-23之间的整数):").strip()
if not start_time.isdigit() or int(start_time) < 0 or int(start_time) > 23:
print("输入的时间不在有效范围内,请重新输入!")
continue
start_time = int(start_time)
# 输入持续时间
duration = input("请输入持续时间(小时,1-24之间的整数):").strip()
if not duration.isdigit() or int(duration) < 1 or int(duration) > 24 or start_time + int(duration) > 24:
print("持续时间不在有效范围内,请重新输入!")
continue
duration = int(duration)
# 检查时间段是否可用
can_book = True
for i in range(duration):
if bookings[day_index][start_time + i]:
can_book = False
break
if can_book:
# 更新预订状态
for i in range(duration):
bookings[day_index][start_time + i] = True
print(f"预订成功!{day.capitalize()}从{start_time}:00到{start_time + duration}:00已预订。")
else:
print(f"预订失败!{day.capitalize()}从{start_time}:00到{start_time + duration}:00时间段已有预订。")
def booking_app():
# 初始化一周的预订状态,7天,每天24小时,默认False表示未预订
bookings = [[False] * 24 for _ in range(7)]
# 定义星期和索引映射
days_of_week = {
"monday": 0,
"tuesday": 1,
"wednesday": 2,
"thursday": 3,
"friday": 4,
"saturday": 5,
"sunday": 6
}
while True:
print("\n欢迎使用预订系统!")
print("请输入需要预订的信息:")
# 输入星期几
day = input("请输入星期几(如:monday, tuesday等,输入'exit'退出):").strip().lower()
if day == 'exit':
print("感谢使用预订系统!")
break
if day not in days_of_week:
print("输入的星期不正确,请重新输入!")
continue
day_index = days_of_week[day]
# 输入起始时间
try:
start_time = int(input("请输入起始时间(24小时制,0-23之间的整数):").strip())
if start_time < 0 or start_time > 23:
print("输入的时间不在有效范围内,请重新输入!")
continue
except ValueError:
print("时间格式不正确,请输入整数!")
continue
# 输入持续时间
try:
duration = int(input("请输入持续时间(小时,1-24之间的整数):").strip())
if duration < 1 or duration > 24 or start_time + duration > 24:
print("持续时间不在有效范围内,请重新输入!")
continue
except ValueError:
print("时间格式不正确,请输入整数!")
continue
# 检查时间段是否可用
can_book = all(not bookings[day_index][start_time + i] for i in range(duration))
if can_book:
# 更新预订状态
for i in range(duration):
bookings[day_index][start_time + i] = True
print(f"预订成功!{day.capitalize()}从{start_time}:00到{start_time + duration}:00已预订。")
else:
print(f"预订失败!{day.capitalize()}从{start_time}:00到{start_time + duration}:00时间段已有预订。")
Question 2
Completion of this question demonstrates competency selection, loops and files, and mastery in selection
Your job is to create a game of MAD-LIBS. Open a file called input.txt and copy the file to output.txt.But you must observe the following rules:
- You should replace all instances of bad words (the only bad words are DARN, HECK, DANG and GOSH[1]) with the word CENSORED
- Every instance of NAME in the file should be replaced with the user’s name (you should only ask them their name once)
- Every instance of the word ACTION in the text should be replaced with an action that the user can specify (you should ask them for a new action each time)
- If the user enters any of the bad words for their name or for actions, you should print the message TSK TSK and instead of the bad word, simply insert the word DUMMY into the output text.
# 定义坏词列表
BAD_WORDS = {"DARN", "HECK", "DANG", "GOSH"} # 定义坏词集合
# 获取用户输入的名字
name = input("请输入你的名字: ").strip() # 提示用户输入名字,并去掉首尾空格
if name.upper() in BAD_WORDS: # 检查名字是否是坏词
print("TSK TSK") # 如果是坏词,提示用户
name = "DUMMY" # 用 "DUMMY" 替代名字
# 打开文件读取内容
infile = open("input.txt", "r", encoding="utf-8") # 打开文件 input.txt,读取模式为 "r",编码为 UTF-8
content = infile.read() # 读取整个文件内容为字符串
infile.close() # 关闭文件以释放资源
# 初始化结果内容列表
output_content = [] # 用于存储处理后的单词列表
# 遍历文件内容逐词替换
for word in content.split(): # 将文件内容按空格分割为单词,并逐一处理
if word == "NAME": # 如果单词是 NAME
word = name # 替换为用户输入的名字
elif word == "ACTION": # 如果单词是 ACTION
action = input("请输入一个动作 (ACTION): ").strip() # 提示用户输入一个动作,并去掉首尾空格
if action.upper() in BAD_WORDS: # 检查用户输入的动作是否是坏词
print("TSK TSK") # 如果是坏词,提示用户
action = "DUMMY" # 用 "DUMMY" 替代动作
word = action # 将单词替换为用户输入的动作
elif word.upper() in BAD_WORDS: # 如果单词是坏词
word = "CENSORED" # 替换为 "CENSORED"
output_content.append(word) # 将处理后的单词添加到结果内容列表
# 写入到 output.txt
outfile = open("output.txt", "w", encoding="utf-8") # 打开文件 output.txt,写入模式为 "w",编码为 UTF-8
outfile.write(" ".join(output_content)) # 将处理后的单词列表连接成字符串并写入文件
outfile.close() # 关闭文件以释放资源
print("处理完成,结果已写入 output.txt") # 提示用户处理完成
# 定义坏词列表
BAD_WORDS = {"DARN", "HECK", "DANG", "GOSH"}
# 获取用户输入的名字
name = input("请输入你的名字: ").strip()
if name.upper() in BAD_WORDS:
print("TSK TSK")
name = "DUMMY"
# 打开文件读取
try:
with open("input.txt", "r", encoding="utf-8") as infile:
content = infile.read()
except FileNotFoundError:
print("文件 input.txt 未找到!")
exit()
# 初始化结果内容列表
output_content = []
# 遍历文件内容逐词替换
for word in content.split():
if word == "NAME": # 替换 NAME
word = name
elif word == "ACTION": # 替换 ACTION
action = input("请输入一个动作 (ACTION): ").strip()
if action.upper() in BAD_WORDS:
print("TSK TSK")
action = "DUMMY"
word = action
elif word.upper() in BAD_WORDS: # 替换坏词为 CENSORED
word = "CENSORED"
# 将替换后的单词添加到结果列表
output_content.append(word)
# 写入到 output.txt
with open("output.txt", "w", encoding="utf-8") as outfile:
outfile.write(" ".join(output_content))
print("处理完成,结果已写入 output.txt")
# 定义坏词列表
BAD_WORDS = {"DARN", "HECK", "DANG", "GOSH"}
def replace_bad_words(word):
"""替换坏词为CENSORED"""
return "CENSORED" if word in BAD_WORDS else word
# 获取用户输入的名字
name = input("请输入你的名字: ").strip()
if name.upper() in BAD_WORDS:
print("TSK TSK")
name = "DUMMY"
# 打开文件读取
try:
with open("input.txt", "r", encoding="utf-8") as infile:
content = infile.read()
except FileNotFoundError:
print("文件 input.txt 未找到!")
exit()
output_content = []
# 替换内容
for word in content.split():
if word == "NAME":
word = name
elif word == "ACTION":
action = input("请输入一个动作 (ACTION): ").strip()
if action.upper() in BAD_WORDS:
print("TSK TSK")
action = "DUMMY"
word = action
else:
word = replace_bad_words(word.upper())
output_content.append(word)
# 写入到 output.txt
with open("output.txt", "w", encoding="utf-8") as outfile:
outfile.write(" ".join(output_content))
print("处理完成,结果已写入 output.txt")
公众号:AI悦创【二维码】
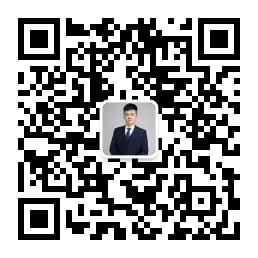
AI悦创·编程一对一
AI悦创·推出辅导班啦,包括「Python 语言辅导班、C++ 辅导班、java 辅导班、算法/数据结构辅导班、少儿编程、pygame 游戏开发、Web、Linux」,全部都是一对一教学:一对一辅导 + 一对一答疑 + 布置作业 + 项目实践等。当然,还有线下线上摄影课程、Photoshop、Premiere 一对一教学、QQ、微信在线,随时响应!微信:Jiabcdefh
C++ 信息奥赛题解,长期更新!长期招收一对一中小学信息奥赛集训,莆田、厦门地区有机会线下上门,其他地区线上。微信:Jiabcdefh
方法一:QQ
方法二:微信:Jiabcdefh
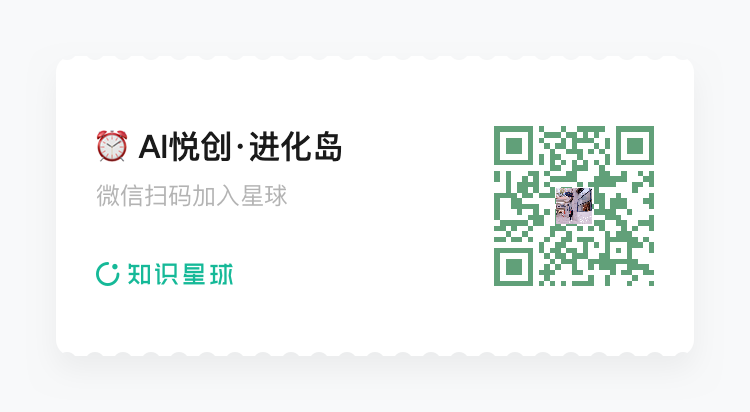
if you know of any others, feel free to add them... but I cant imagine a good university student like you knows any worse words ↩︎